Create Real Payment
Through the createPayment
action, payments with actual cryptocurrencies can be created.
You can enable / disabled a cryptocurrency or a specific network within your dashboard.
How payments works?
1. The user will access the payment link using the generated paymentID
returned by our API.
2. They can choose their preferred cryptocurrency and network (in those you accept).
3. They will be prompted to transfer the required amount to a designated address.
4. Once the required amount has been received, we will send a webhook
request to your webhook endpoint in order to notify the success of the payment (alternatively, you can use the getPaymentStatus
API to check the payment status).
5. Subsequently, the funds will be automatically transferred to your desired wallet.
Requirements
In order to create a payment, you will need:
- Your API Token:
abcd...
which can be found in your dashboard here. - The action:
createPayment
- Your desired amount in dollars:
10
It's crucial to keep your API Token confidential. Tokens should never be exposed in front-end code. It's advised to make the API request server-side to prevent parameter tampering and token leaks.
The HTTPS Request
To initiate a payment, make a GET request to https://api.swaycoin.io, including the required parameters.
https://api.swaycoin.io?token=YOUR_API_TOKEN&action=createPayment&amount=10
Using Code
- Node.js
- Python
- PHP
const YOUR_API_TOKEN = 'YOUR_API_TOKEN';
const amount = 10;
const endpoint = 'https://api.swaycoin.io?token=' + YOUR_API_TOKEN + '&action=createPayment&amount=' + amount;
fetch(endpoint)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
import requests
YOUR_API_TOKEN = 'YOUR_API_TOKEN'
amount = 10
endpoint = f"https://api.swaycoin.io?token={YOUR_API_TOKEN}&action=createPayment&amount={amount}"
response = requests.get(endpoint)
data = response.json()
print(data)
<?php
$YOUR_API_TOKEN = 'YOUR_API_TOKEN';
$amount = 10;
$endpoint = 'https://api.swaycoin.io?token=' . $YOUR_API_TOKEN . '&action=createPayment&amount=' . $amount;
$response = file_get_contents($endpoint);
echo $response;
?>
The HTTPS Response
Upon successful execution, you should receive a JSON response similar to:
// Response indicating a successful request
{
"request_status": "success",
"message": "Your payment has been successfully created!",
"paymentID": "0123456789"
}
Upon failed execution, you should receive a JSON response similar to:
// Response indicating a failed request
{
"request_status": "error",
"message": "Invalid API token, action, or amount. Please check your input parameters."
}
A valid paymentID
for real payments will consist of 10 numbers only.
Forwarding the user
To proceed with the payment, redirect the user to https://payment.swaycoin.io, appending the newly generated paymentID
as the paymentID parameter.
URL Example:
// Replace 0123456789 by the paymentID received within the response
https://payment.swaycoin.io?paymentID=0123456789
SwayCoin.io Payment Website
Please select your currency for your $10 Payment.
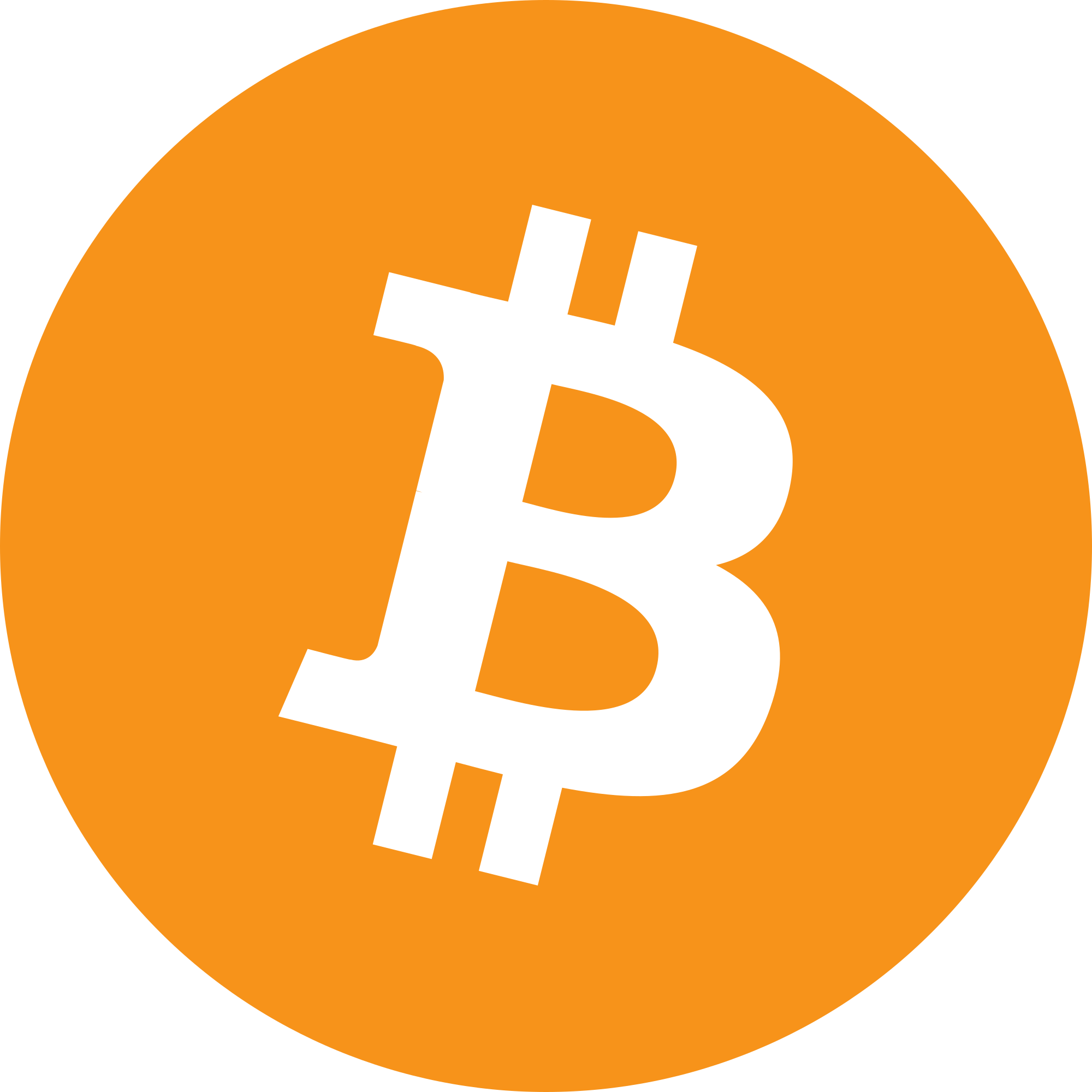
BTC
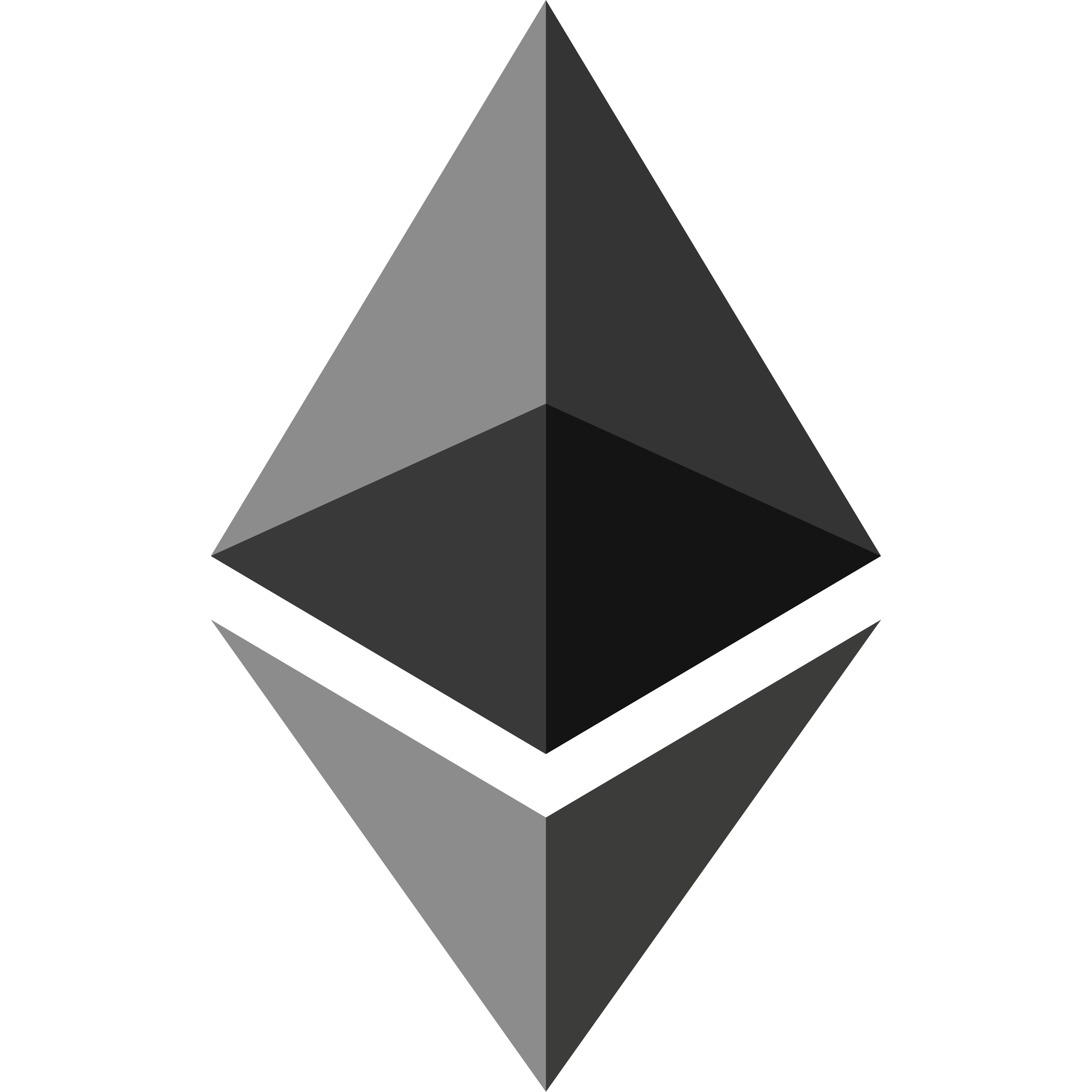
ETH
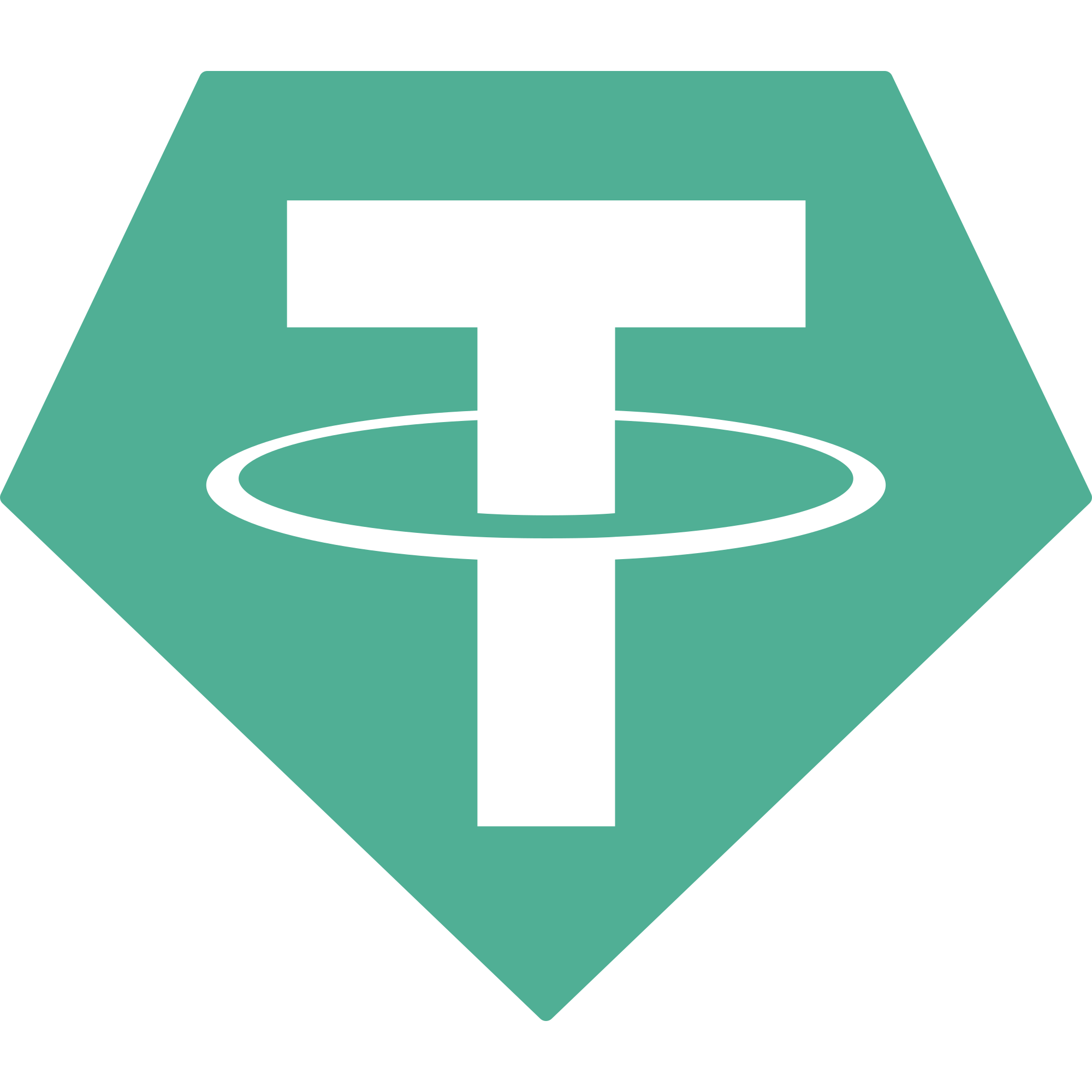
USDT