Create Test Payment
Creating a Test Payment (createTestPayment
) allows you to verify the functionality of your webhook endpoint, token, parameters, and all necessary informations.
This process closely mimics a real payment. However, note that you can only pay using faucets within the Sepolia Test Network (Native SepoliaETH Coin & ERC-20 Faucet Tokens).
How to obtain these faucets?
Requirements
In order to create a test payment, you will need:
- Your API Token:
abcd...
which can be found in your dashboard here. - The action:
createTestPayment
- Your desired amount in dollars:
10
It's crucial to keep your API Token confidential. Tokens should never be exposed in front-end code. It's advised to make the API request server-side to prevent parameter tampering and token leaks.
The HTTPS Request
To initiate a test payment, make a GET request to https://api.swaycoin.io, including the required parameters.
https://api.swaycoin.io?token=YOUR_API_TOKEN&action=createTestPayment&amount=10
Using Code
- Node.js
- Python
- PHP
const YOUR_API_TOKEN = 'YOUR_API_TOKEN';
const amount = 10;
const endpoint = 'https://api.swaycoin.io?token=' + YOUR_API_TOKEN + '&action=createTestPayment&amount=' + amount;
fetch(endpoint)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
import requests
YOUR_API_TOKEN = 'YOUR_API_TOKEN'
amount = 10
endpoint = f"https://api.swaycoin.io?token={YOUR_API_TOKEN}&action=createTestPayment&amount={amount}"
response = requests.get(endpoint)
data = response.json()
print(data)
<?php
$YOUR_API_TOKEN = 'YOUR_API_TOKEN';
$amount = 10;
$endpoint = 'https://api.swaycoin.io?token=' . $YOUR_API_TOKEN . '&action=createTestPayment&amount=' . $amount;
$response = file_get_contents($endpoint);
echo $response;
?>
The HTTPS Response
Upon successful execution, you should receive a JSON response similar to:
// Response indicating a successful request
{
"request_status": "success",
"message": "Your payment has been successfully created!",
"paymentID": "XXXXXXXXXX"
}
Upon failed execution, you should receive a JSON response similar to:
// Response indicating a failed request
{
"request_status": "error",
"message": "Invalid API token, action, or amount. Please check your input parameters."
}
A valid paymentID
for test payments will consist of 10 letters only.
Forwarding the user
To proceed with the payment, redirect the user to https://payment.swaycoin.io, appending the newly generated paymentID
as the paymentID parameter.
URL Example:
// Replace XXXXXXXXXX by the paymentID received within the response
https://payment.swaycoin.io?paymentID=XXXXXXXXXX
SwayCoin.io Test Payment Website
Please select your currency for your $10 Payment.
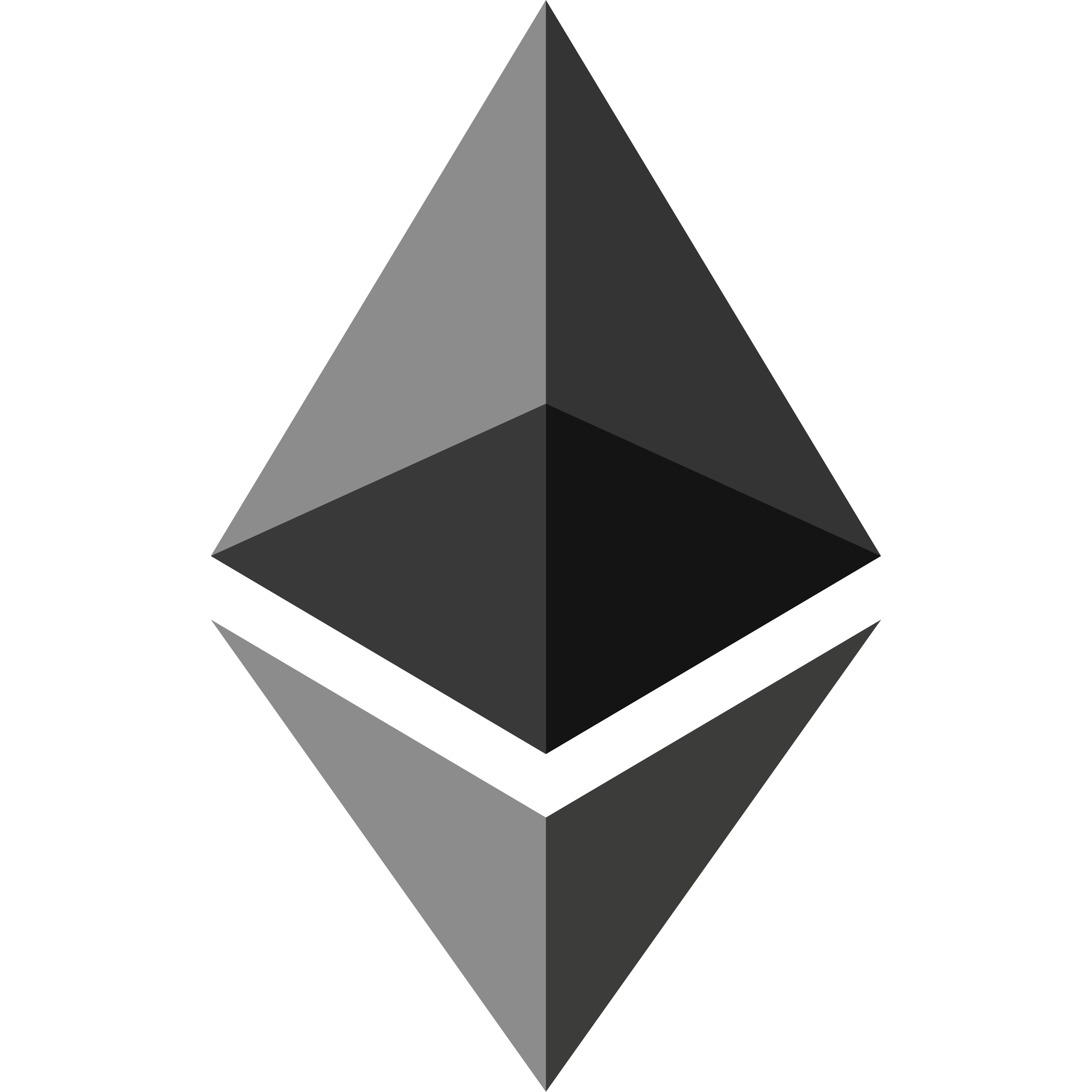
SepoliaETH